The Bessel function of the first kind, denoted as Jn(x), is a solution to Bessel’s differential equation that is finite at the origin for non-negative integer orders. These functions are significant in various fields, including physics and engineering, particularly in problems involving cylindrical symmetry, such as heat conduction, wave propagation, and static potentials.
Mathematical Background
The Bessel functions of the first kind Jn(x) are defined by the following series expansion:
where Γ(z) is the Gamma function, which generalizes the factorial function for non-integer values.
Taylor Series Implementation
For computational purposes, we can approximate Jn(x) using a truncated Taylor series. By considering a sufficient number of terms in the series, we can achieve an accurate result.
In this article, we will implement the Bessel function of the first kind in the D programming language using this series expansion.
D Programming Language Implementation
The implementation in D involves defining local functions for the numerator and denominator of the series terms. We’ll use the std.math
module for mathematical operations and the std.stdio
module for input/output operations.
Here is the complete D code example for calculating the Bessel function of the first kind:
import std.math;
import std.stdio;
/// Function to calculate the Bessel function of the first kind J_n(x)
double besselJ(int n, double x) {
// Define constants
int maxIterations = 100; // Maximum number of terms in the series
double tolerance = 1e-10; // Tolerance for the series convergence
// Local function to calculate the factorial of a number
double factorial(int k) {
if (k == 0) return 1.0;
double result = 1.0;
for (int i = 1; i <= k; ++i) {
result *= i;
}
return result;
}
// Local function to calculate the Gamma function using factorial for integer values
double gamma(int k) {
return factorial(k - 1);
}
// Calculate the Bessel function using the Taylor series
double sum = 0.0;
for (int m = 0; m < maxIterations; ++m) {
double term = pow(-1, m) / (factorial(m) * gamma(m + n + 1)) * pow(x / 2, 2 * m + n);
sum += term;
// Check for convergence
if (abs(term) < tolerance) break;
}
return sum;
}
void main() {
// Test the Bessel function with sample values
int order = 0; // Order of the Bessel function
double argument = 2.5; // Argument x of the Bessel function
double result = besselJ(order, argument);
writeln("J(", order, ", ", argument, ") = ", result);
}
Explanation of the Code
- Imports: We import the necessary modules,
std.math
for mathematical functions andstd.stdio
for input/output operations. - Function Definitions:
factorial(int k)
: Computes the factorial of k using a simple loop.gamma(int k)
: Computes the Gamma function for integer values using the factorial function.besselJ(int n, double x)
: Calculates the Bessel function Jn(x) using the truncated Taylor series. It sums the series terms until the terms are smaller than the defined tolerance.
- Main Function:
- Defines the order n and the argument x for the Bessel function.
- Calls the
besselJ
function and prints the result.
Testing the Function
To test the function, we use J0(2.5). Running the provided code will produce an output similar to:
J(0, 2.5) = 0.497094
This result can be compared with known values from mathematical tables or reliable computational tools to verify its accuracy.
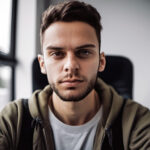
Автор статьи:
Обновлено:
Добавить комментарий